Introduction
In this guide, we’ll explore the creation of a Fake Sales Notification system using HTML, CSS, and JavaScript. This system simulates real-time purchase alerts, adding a dynamic and engaging element to your website. We’ll dive into the code, dissecting each component’s functionality, and provide insights into how you can customize this feature for your website.
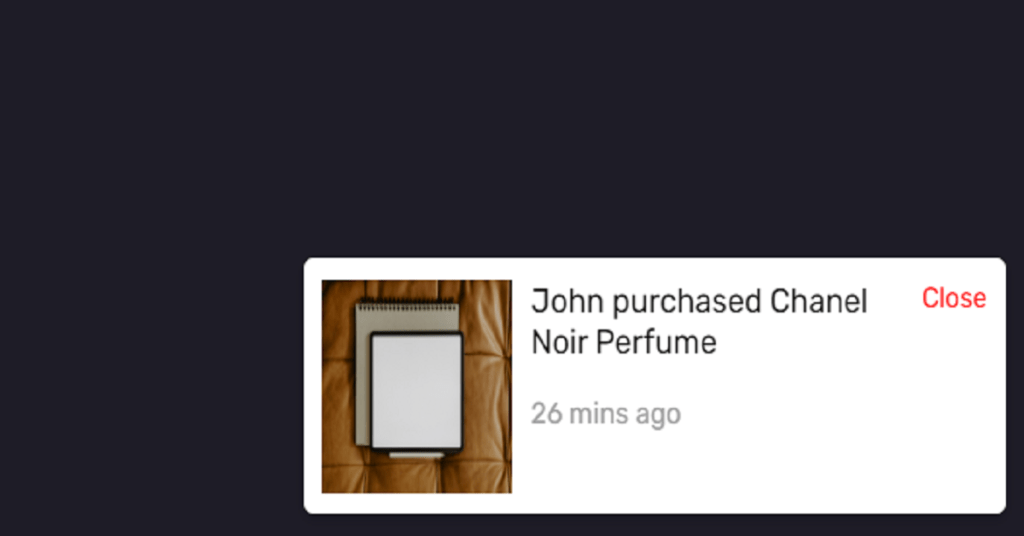
HTML Structure
<!DOCTYPE html>
<html lang="en">
<head>
<meta charset="UTF-8" />
<meta name="viewport" content="width=device-width, initial-scale=1.0" />
<title>Fake Sales Notification</title>
<link
href="https://fonts.googleapis.com/css2?family=Rubik:wght@400;600&display=swap"
rel="stylesheet"
/>
</head>
<body>
<div id="custom-notification">
<div class="custom-product-info">
<img src="" alt="Product Image" id="custom-product-image" />
<div id="custom-product-text"></div>
</div>
<button id="custom-close-btn">Close</button>
</div>
</body>
</html>
HTMLHTML Breakdown:
- Notification Container: A fixed-position container at the bottom right, initially hidden.
- Product Information: Displays the product image and purchase information.
- Close Button: Allows users to close the notification.
CSS Styling
* {
margin: 0;
padding: 0;
font-family: "Rubik", sans-serif;
}
body {
background-color: #1e1c27;
}
#alert-btn {
cursor: pointer;
}
#custom-notification {
width: 350px;
position: fixed;
bottom: 20px;
right: 20px;
padding: 10px;
border-radius: 5px;
box-shadow: 0 2px 5px rgba(0, 0, 0, 0.2);
display: none;
align-items: flex-start;
gap: 0.5em;
background-color: white;
}
.custom-product-info {
display: flex;
}
.custom-product-info img {
width: 100px;
height: 100px;
margin-right: 10px;
}
.custom-message {
font-size: 16px;
}
.custom-time {
font-size: 14px;
margin-top: 16px;
color: #a0a0a0;
}
#custom-close-btn {
background-color: transparent;
color: red;
border: none;
outline: none;
cursor: pointer;
}
CSSCSS Highlights:
- Global Styles: Resets margin, padding, and sets the font family.
- Notification Styles: Defines appearance, position, and shadow for the notification.
- Product Info Styles: Styles for the product image and text display.
- Close Button Styles: Styles for the close button.
JavaScript Logic
const customNotification = document.getElementById("custom-notification");
const closeNotificationBtn = document.getElementById("custom-close-btn");
const customProductText = document.getElementById("custom-product-text");
const customProductImage = document.getElementById(
"custom-product-image"
);
const names = ["John", "Alice", "Bob", "Emma", "Betty"];
const products = [
{
name: "Nike Red Shoes",
image: "card-1-img.jpg",
},
{
name: "Chanel Noir Perfume",
image: "card-2-img.jpg",
},
{
name: "Awesome Black Shirt",
image: "card-3-img.jpg",
},
{
name: "Ray-Ban Sunglasses",
image: "sun.png",
},
{
name: "Black Apple Watch",
image: "tiger.png",
},
];
function getRandomItemFromArray(arr) {
return arr[Math.floor(Math.random() * arr.length)];
}
function getRandomTime() {
return Math.floor(Math.random() * 59) + 1;
}
const getRandomDisplayTime = () => Math.random() * (8 - 3) + 3;
const showCustomNotification = () => {
const randomName = getRandomItemFromArray(names);
const randomProduct = getRandomItemFromArray(products);
const { name, image } = randomProduct;
customProductImage.src = image;
customProductText.innerHTML = `<p class="custom-message">${randomName} purchased ${name}</p> <p class="custom-time">${getRandomTime()} mins ago</p>`;
customNotification.style.display = "flex";
};
closeNotificationBtn.addEventListener("click", () => {
customNotification.style.display = "none";
setTimeout(
showCustomNotification,
Math.floor(getRandomDisplayTime()) * 500
);
});
setTimeout(
showCustomNotification,
Math.floor(getRandomDisplayTime()) * 500
);
JavaScriptJavaScript Breakdown:
- Element Selection: Selects notification elements and initializes variables.
- Data Definition: Defines arrays for names and products with respective images.
- Randomization Functions: Functions for obtaining random items from arrays and generating random times.
- Display Function: Displays a fake sales notification with a random name, product, and time.
- Event Listener: Closes the notification and schedules the next display after a set time.
Conclusion
By implementing this Fake Sales Notification system, you can create a sense of activity and user engagement on your website. Customize the names, products, and appearance to suit your brand’s style. Feel free to experiment with additional features or integrate it with your backend to show real-time data.
Happy Coding!